A print-in-place single-fold hinge generator.
- Parameters
-
wth | <decimal> wall thickness. |
size | <datastruct | decimal> pivot and plate sizes; a list [l, w], the lengths and widths or a single decimal to set the lengths. The parameter l is a <decimal list-3 | decimal> [lh, lpl, lpr], the hinge, left, and right plate lengths or a single decimal to set (lh=lpl=lpr). Parameter w is a <decimal-list-2 | decimal> [wpl, wpr], the left and right plate widths or a single decimal to set (wpl=wpr). Any unspecified parameters are assigned the last specified value. |
vr | <datastruct | decimal> plate rounding radii; a list [vrl, vrr], the left and right rounding radii or a single decimal to set (vrl=vrr). The parameters vrl and vrr are <decimal-list-4 | decimal>, the corner rounding radii for the left and right plates or a single decimal to round all corners the same. |
vrm | <datastruct | integer> plate rounding mode; a list [vrml, vrmr], the left and right rounding modes or a single integer to set (vrml=vrmr). The parameters vrml and vrmr are <integer-list-4 | integer>, the corner rounding modes for the left and right plates or a single integer to round all corners the same. |
knuckle | <datastruct> knuckles (see below). |
offset | <decimal-list-2 | dcimal> pivot-to-plate offset; a list [oz, oy], the z-offset and y-offset between the pivot and plates or a single decimal to set oz . The default offset value is [-wth/2, 0] for a backflap hinge configuration. |
pbore | <datastruct> the knuckle pivot bore (see below). |
mbore | <datastruct> mount plate bore (see below). |
mbores | <datastruct> mount plate bore instance list (see below). |
support | <boolean> add print support for knuckles. |
mode | <integer> part mode (see below). |
pivot | <decimal-list-2 | decimal> the pivot; a list [pl, pr], the left and right mount plate pivots or a single decimal to set (pl=pr). |
align | <integer-list-3 | integer> the part alignment; a list [x, y, z], or a single integer to set z (default = [0, 0, 0]). |
verb | <integer> console output verbosity {0=quiet, 1=info}. |
This module constructs custom single-fold hinges; such as a butt, backflap, strap, gate, offset, continuous, or a knuckle hinge. Most aspects of the hinge can be controlled via a parameter and the base configuration is for a version that can be printed in-place on a 3d printer. Alternatively, the pivot bore can be specified for use with metal pins. The plate size and mount holes are configurable and the bores are generated by screw_bore().
Multi-value and structured parameters
knuckle
Data structure fields: knuckle
e | data type | default value | parameter description |
0 | <decimal> | wth | kd: diameter |
1 | <integer> | 2 | count |
2 | <decimal> | 1/2 | gap |
3 | <integer> | 0 | mode |
4 | <datastruct> | 4 | pin |
Data structure fields: knuckle[3]: mode
v | description |
0 | cylinder with no gap |
1 | male pin |
2 | female pin |
3 | gaped cylinders |
4 | hole for external pin |
5 | print support projection (for internal use) |
Data structure fields: knuckle[4]: pin
e | data type | default value | parameter description |
0 | <decimal> | kd*3/4 | pd: cone base diameter |
1 | <decimal> | pd/2 | cone height |
2 | <decimal> | pd/3 | point rounding radius |
pbore, mbore
The hinge pivot and the mount plate bores use a common specification and are performed using screw_bore(). The data structure is detailed in the following section.
Data structure fields: pbore, mbore
e | data type | default value | parameter description |
0 | <decimal> | 0 | bd: bore diameter |
1 | <datastruct> | undef | bh: bore head |
2 | <datastruct> | undef | bn: bore nut |
3 | <datastruct> | 1 | bf: bore scale factor |
The documentation of the bore parameters bh
, bn
and bf
can be found in the screw_bore().
mbores
Data structure schema:
name | schema |
mbores | [ instances ] |
instances | [ instance, instance, ..., instance ] |
mbores[0]: instances
e | data type | default value | parameter description |
0 | <datastruct-list> | undef | instance list |
Data structure fields: mbores[0]: instance
e | data type | default value | parameter description |
0 | <decimal-list-2 | decimal> | required | bore center offset [x, y] |
For an instance with a single decimal, the supplied value controls the bore instance x-offset
(with y=0).
part mode
A binary encoded integer value.
b | description |
0 | generate left mount plate |
1 | generate right mount plate |
Backflap hinge example script
include <omdl-base.scad>;
include <models/3d/fastener/screws.scad>;
include <parts/3d/fastener/hinges.scad>;
$fn = 36;
for (y = [[1, -2], [2, +2]])
(
wth=3,
size=[[28,30], 10],
vr=1,vrm=1,
pivot=45/2,
);
function second(v)
Return the second element of an iterable value.
function first(v)
Return the first element of an iterable value.
module hinge_sf(wth=1, size, vr, vrm, knuckle, offset, pbore, mbore, mbores, support=true, mode=3, pivot, align, verb=0)
A print-in-place single-fold hinge generator.
Backflap hinge example diagramtop | bottom | right | diag |
---|
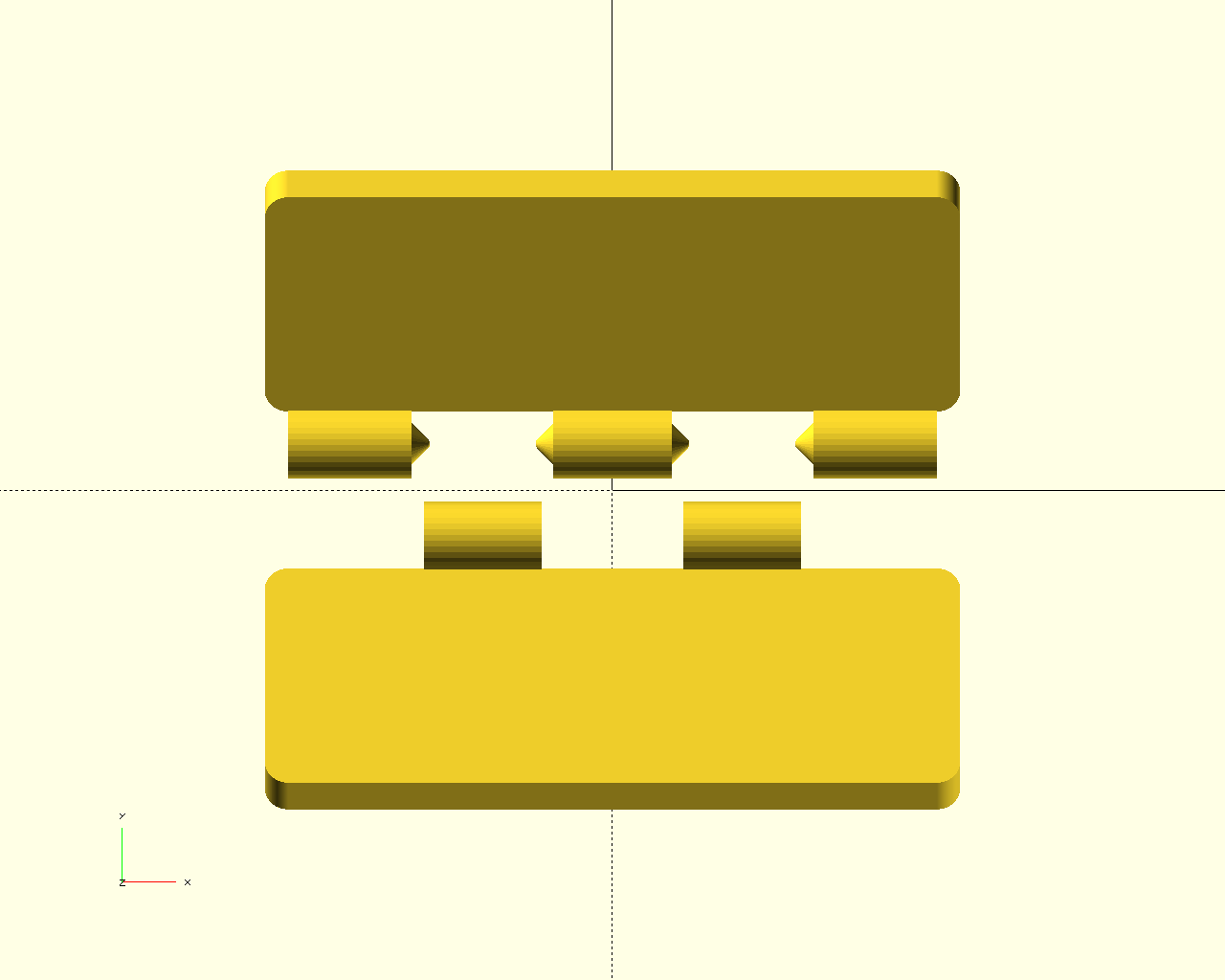 | 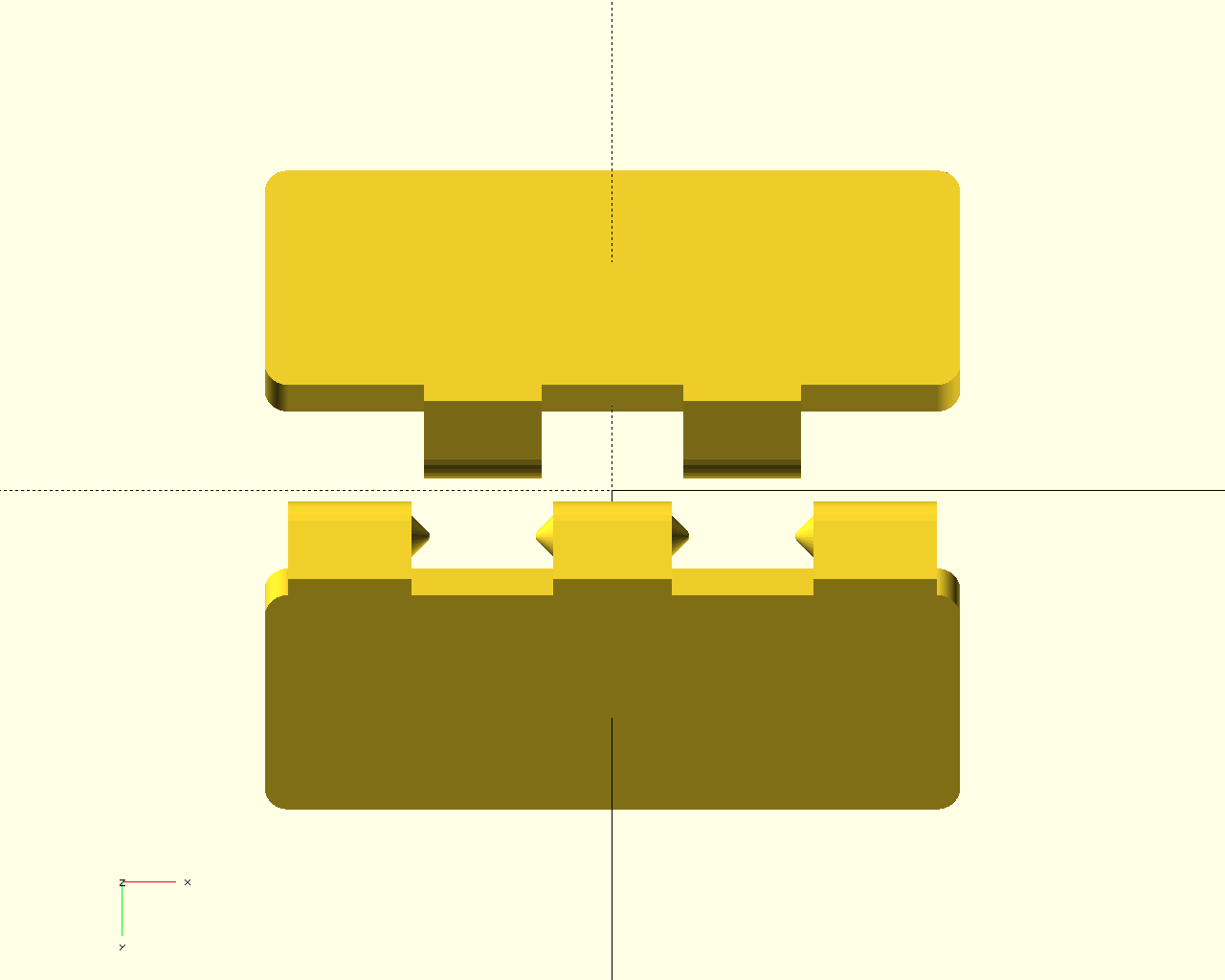 | 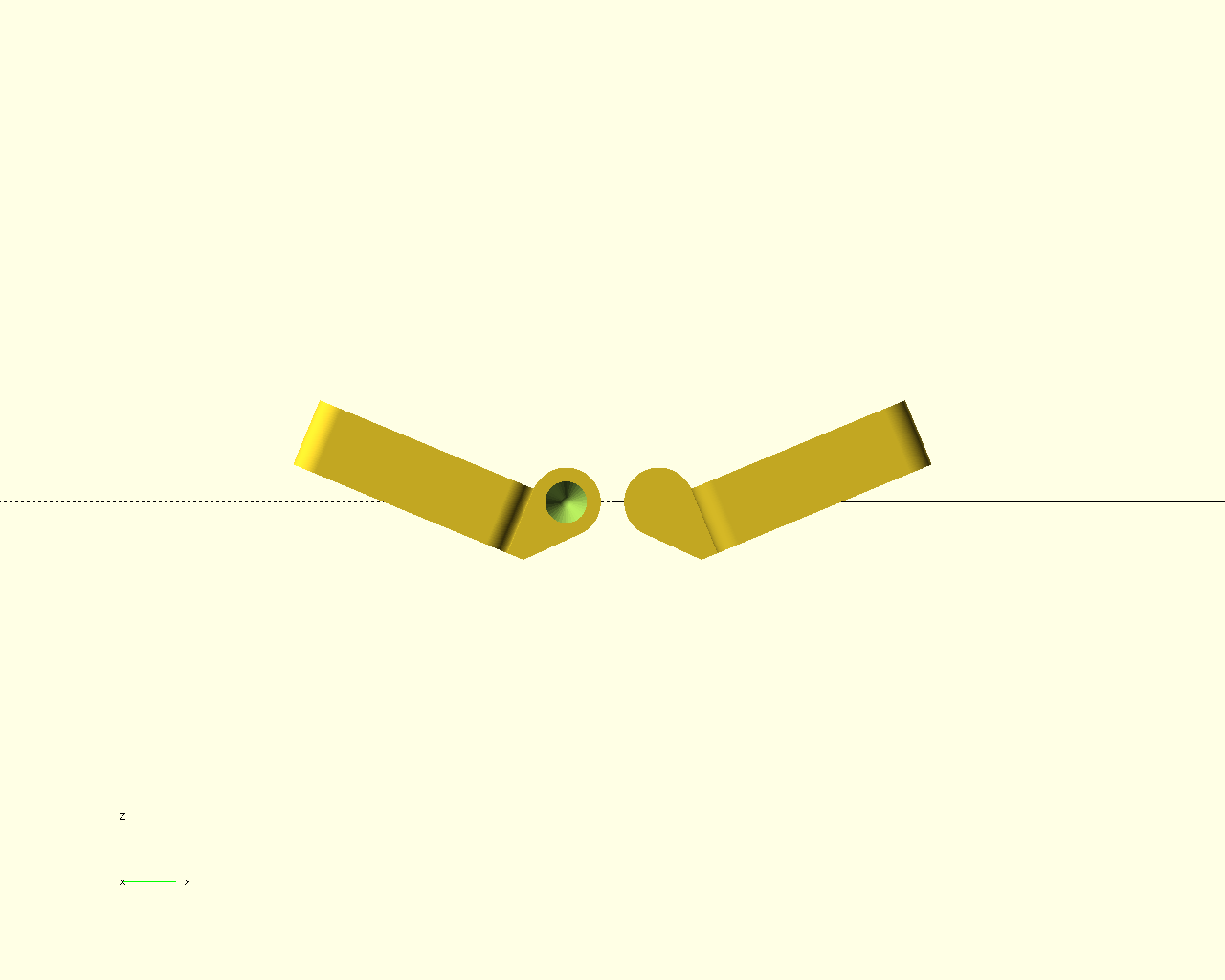 | 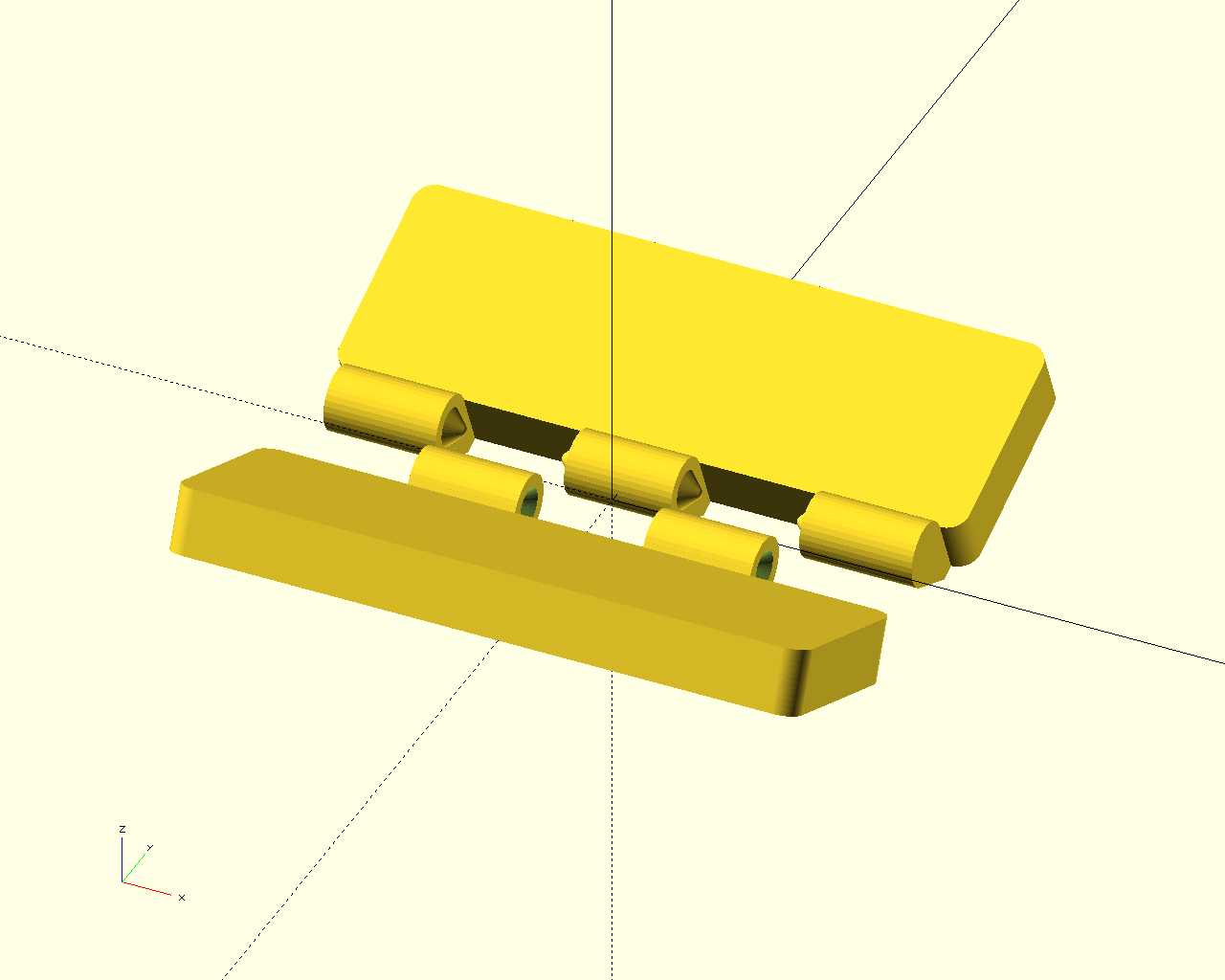 |
Custom hinge example script
include <omdl-base.scad>;
include <models/3d/fastener/screws.scad>;
include <parts/3d/fastener/hinges.scad>;
$fn = 36;
for (y = [[1, -3], [2, +3]])
(
wth=3,
size=[[18, 20, 35], [4, 6]],
vr=1,
vrm=[[4,3,1,1],[10,9,5,5]],
knuckle=[5, 2, 1/2],
mbore=[1, [2, 1, 1/2], [2, 1]],
mbores=[[-7, 0, 7], [-14, -7, 0, 7, 14]],
offset=[undef, 2],
);
Custom hinge example diagramtop | bottom | right | diag |
---|
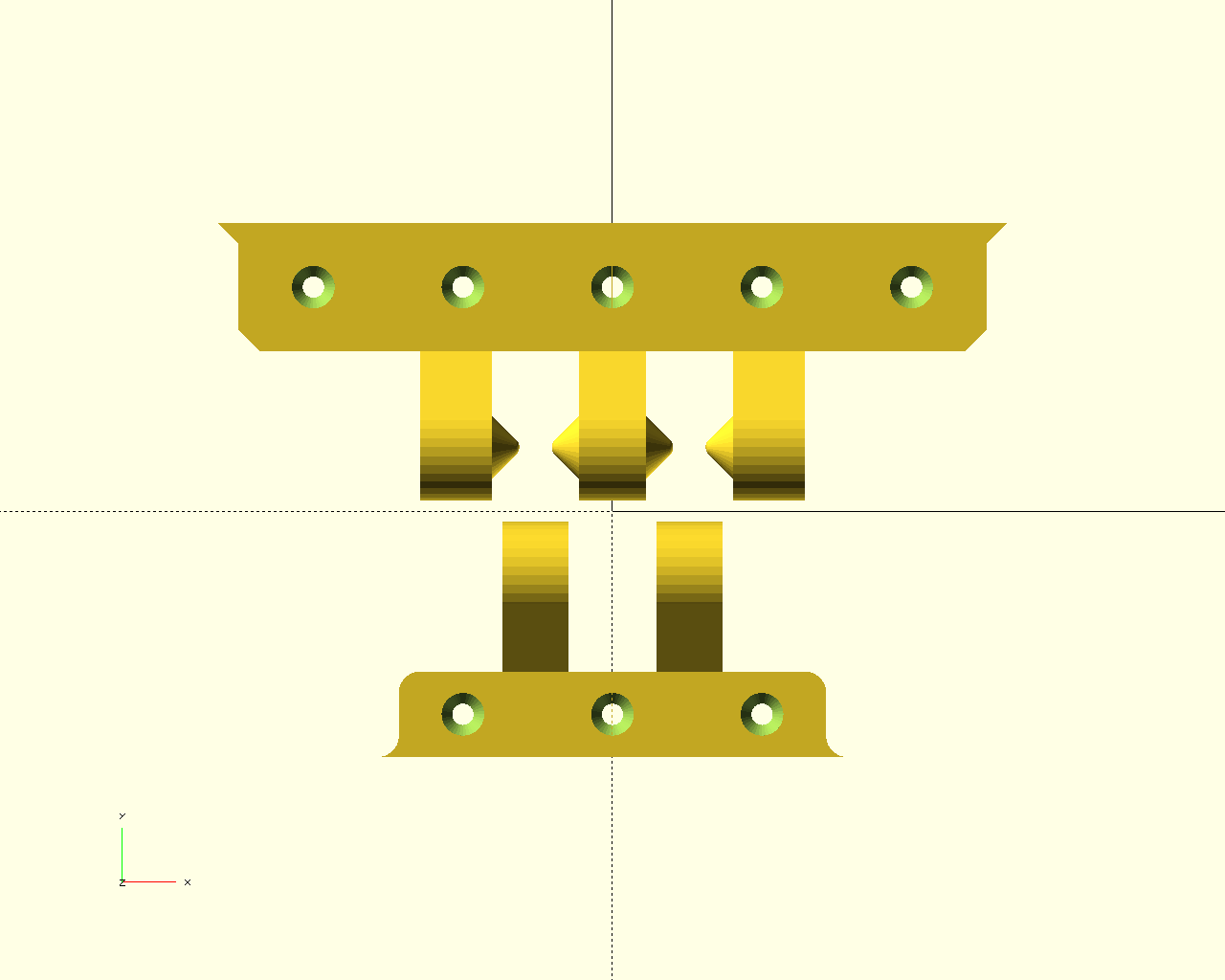 | 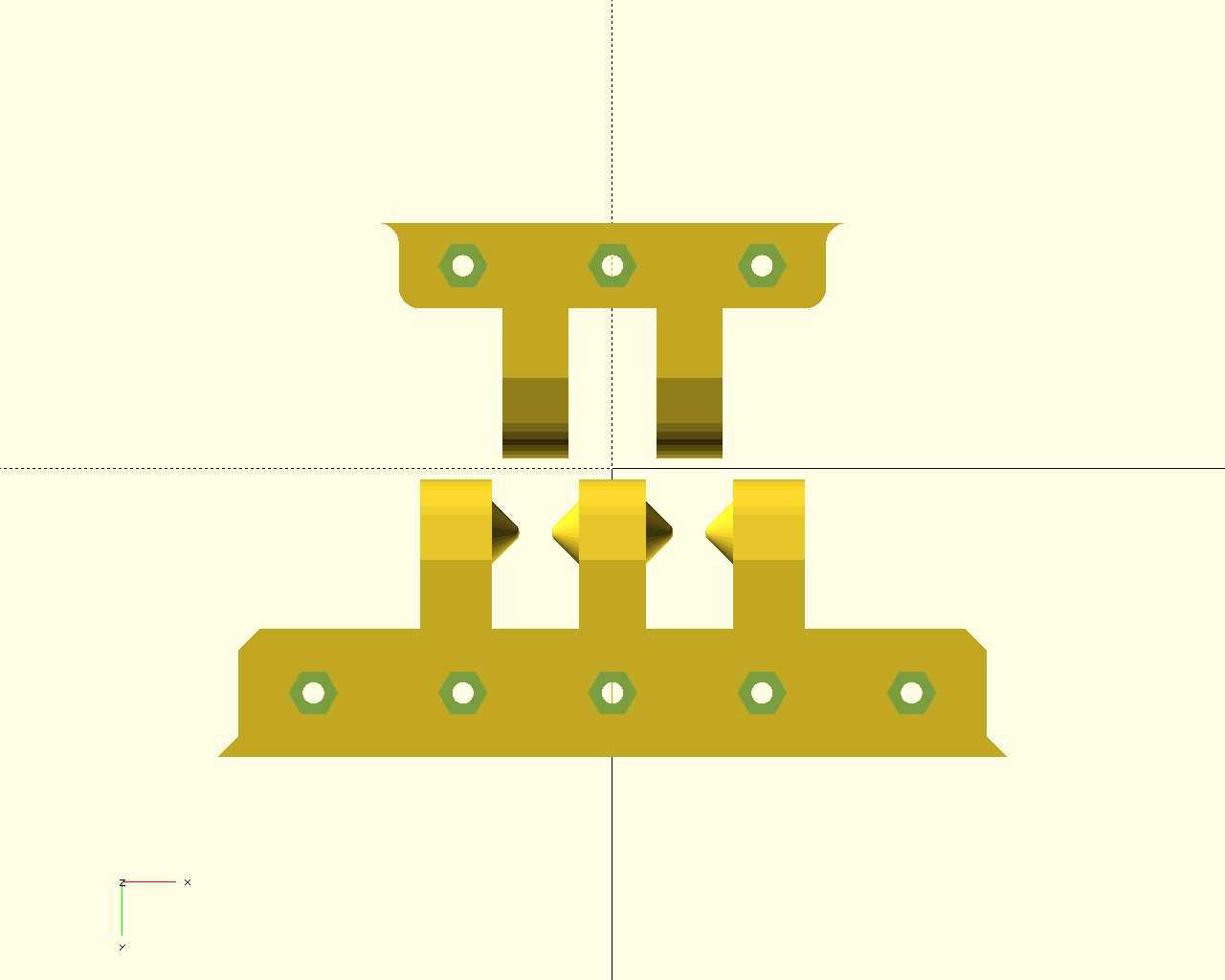 | 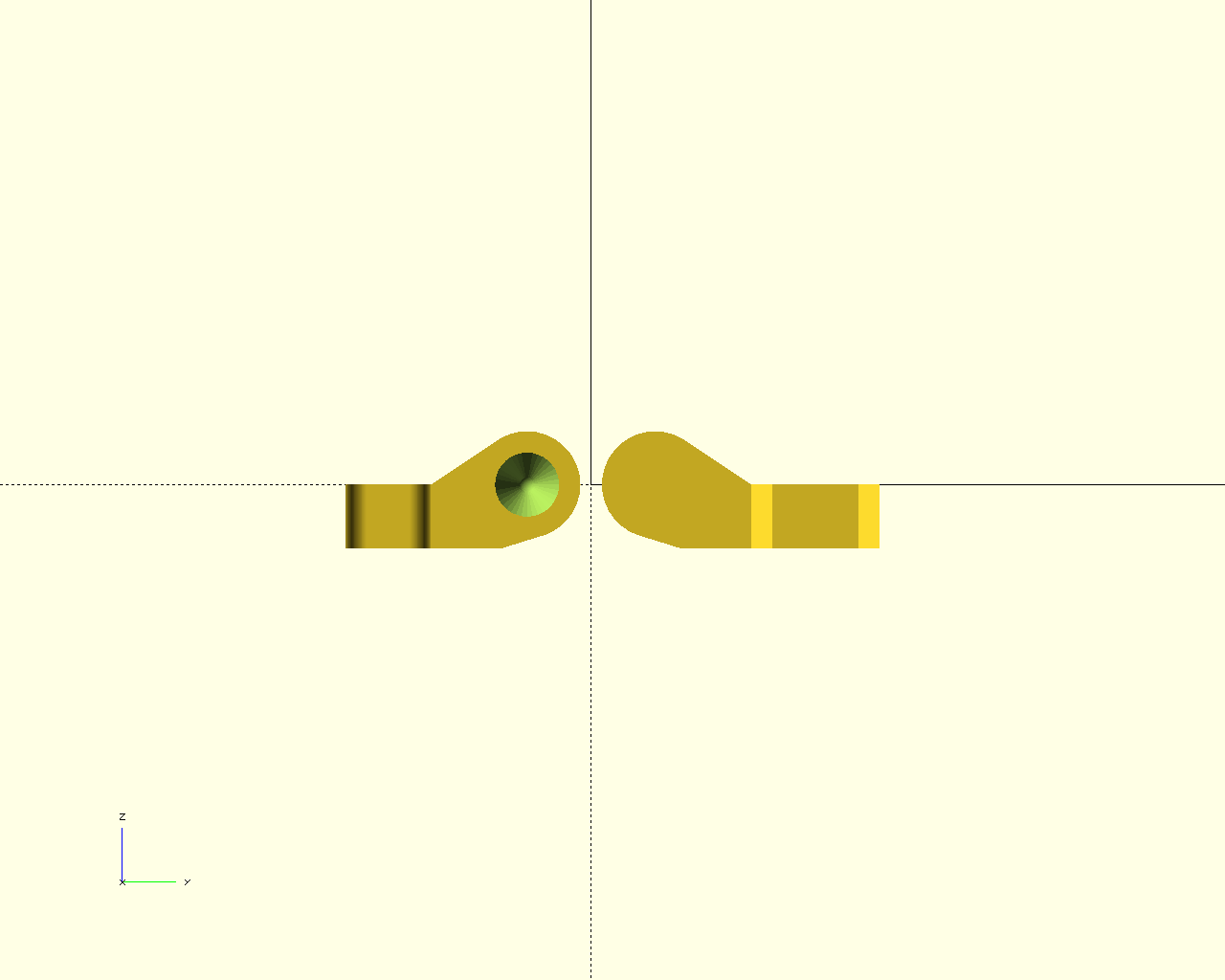 | 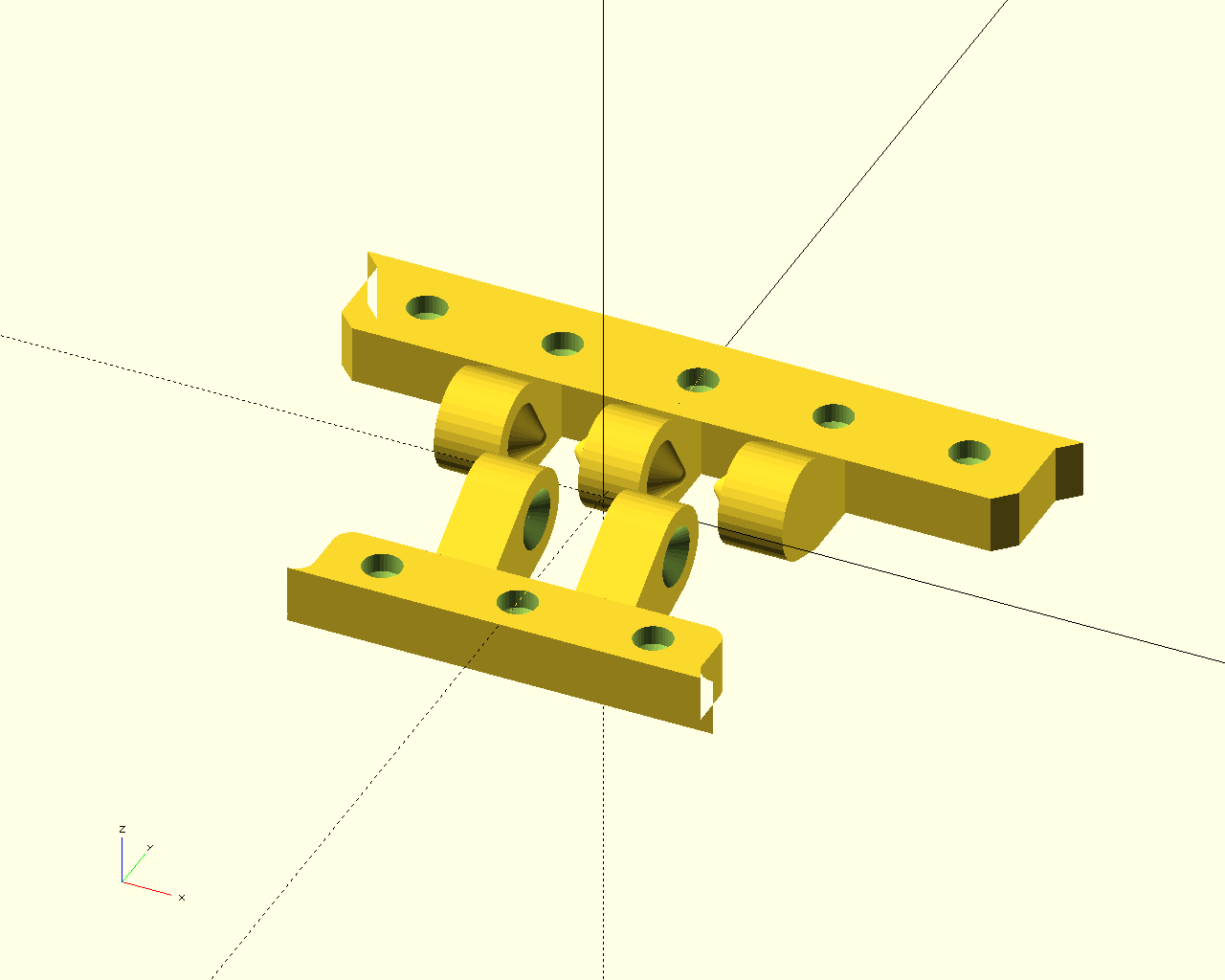 |
Definition at line 635 of file hinges.scad.